在学习linux系统编程的时候,实现了rmdir命令的特别版本。
因为rmdir只能删除空文件夹,而我实现的功能相当于 rm -rf path…
实现的功能
递归删除指定文件夹的所有文件
程序说明
1. my_rmdir(): 即为递归删除动作的自定义函数。
2. opendir(), readdir(), closedir(): 读取目录信息。
3. rmdir(): 删除空的文件夹; remove(): 删除文件或文件夹。
程序编译运行
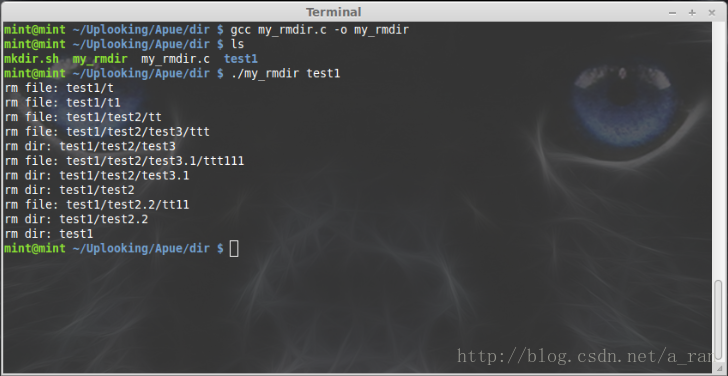
程序源码
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
| #include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <errno.h>
#include <sys/types.h>
#include <dirent.h>
#include <unistd.h>
#define PATH_SIZE 4094
void my_rmdir(const char * path);
/* rm -rf path: ./a.out path */
int main(int argc, char const *argv[])
{
if (argc != 2)
{
fprintf(stdout, "argument error!\n");
return 1;
}
my_rmdir(argv[1]);
return 0;
}
void my_rmdir(const char * path)
{
DIR *dirp;
dirp = opendir(path);
if (NULL == dirp)
{
perror(path);
return;
}
struct dirent *entry;
int ret;
while (1)
{
entry = readdir(dirp);
if (NULL == entry)
{
break;
}
// skip . & ..
if (0 == strcmp(".", entry->d_name) || 0 == strcmp("..", entry->d_name))
{
continue;
}
char buf[PATH_SIZE];
snprintf(buf, PATH_SIZE, "%s/%s", path, entry->d_name);
ret = remove(buf);
if (-1 == ret)
{
if (ENOTEMPTY == errno)
{
my_rmdir(buf);
continue;
}
perror(buf);
return;
}
fprintf(stdout, "rm file: %s\n", buf);
}
closedir(dirp);
ret = rmdir(path);
if (-1 == ret)
{
perror(path);
return;
}
fprintf(stdout, "rm dir: %s\n", path);
}
|